[toc]
python 日常练习
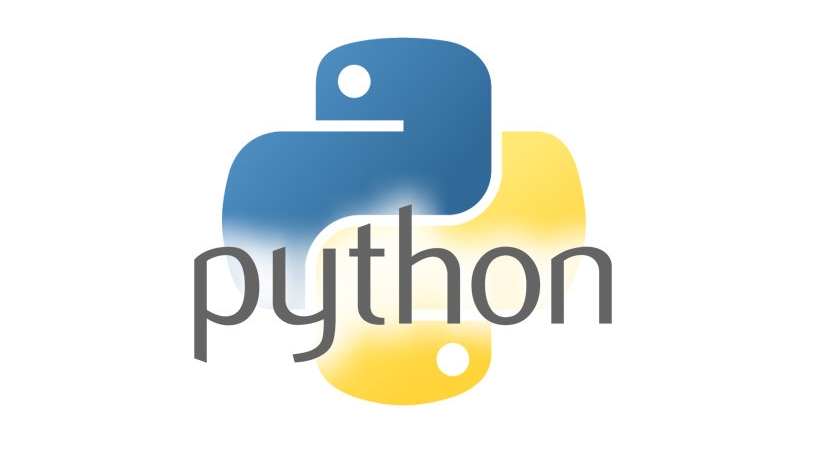
解压序赋值给多个变量
现有一个包含N个元素的元组或者是序列,将它里面的值解压后同时赋值给N个变量
| In [22]: p = (1,2) |
| In [23]: a,b = p |
| In [24]: a |
| Out[24]: 1 |
| In [25]: b |
| Out[25]: 2 |
| |
| |
| In [26]: date = ['test','frum','bind','lance',(201,202,203)] |
| In [27]: te,fr,bi,la,data = date |
| In [28]: te |
| Out[28]: 'test' |
| In [29]: fr |
| Out[29]: 'frum' |
| In [30]: bi |
| Out[30]: 'bind' |
| In [31]: date |
| Out[31]: ['test', 'frum', 'bind', 'lance', (201, 202, 203)] |
| In [32]: data |
| Out[32]: (201, 202, 203) |
| |
| |
| In [41]: a = 'Hello' |
| In [42]: b,c,d,e,f = a |
| In [43]: b |
| Out[43]: 'H' |
| In [44]: c |
| Out[44]: 'e' |
| In [45]: d |
| Out[45]: 'l' |
| In [46]: f |
| Out[46]: 'o' |
初级练习题
给一个半径,求圆的面积和周长。圆周率3.14
| from math import pi |
| r=int(input('r=')) |
| print('area='+str(pi*r*r)) |
| print('circumference='+str(2*pi*r)) |
| |
| (py3_env) kernel ➤ python test.py |
| r=10 |
| area=314.1592653589793 |
| circumference=62.83185307179586 |
输入两个数,比较大小后,从小到大升序打印
| a = input('first: ') |
| b = input('second: ') |
| |
| if a > b: |
| print(b,a) |
| else: |
| print(a,b) |
| |
| (py3_env) kernel ➤ python test.py |
| first: 10 |
| second: 11 |
| 10 11 |
输入n个数,求每次输入后的算数平均数
| n = 0 |
| sum = 0 |
| |
| while True: |
| i = input(">>>") |
| if i == 'quit': |
| break |
| |
| n += 1 |
| sum += int(i) |
| avg = sum/n |
| print(avg) |
打印九九乘法表
| for i in range(1,10): |
| for j in range(1,i+1): |
| print(str(j)+'x'+ str(i) +"=" +str(i*j),end=' ') |
| |
| |
| for i in range(1,10): |
| for j in range(1,i+1): |
| product = i*j |
| |
| if j > 1 and product <10: |
| product = str(product) + ' ' |
| else: |
| product = str(product) |
| print(str(j)+'x'+str(i)+"="+str(product),end=' ') |
| print() |
打印菱形
| for i in range(-3,4): |
| if i<0: |
| prespace = -i |
| else: |
| prespace = i |
| |
| print(' '*prespace + '*'*(7-prespace*2)) |
斐波那契数列,100以内
| 斐波那契数列:1,1,2,3,5,8,13,21,34,55,89,144,... |
| a = 0 |
| b = 1 |
| |
| while True: |
| c = a+b |
| if c > 100: break |
| a=b |
| b=c |
| print(c) |
| |
| a = 1 |
| b = 1 |
| index = 2 |
| print('{0},{1}'.format(0,0)) |
| print('{0},{1}'.format(1,1)) |
| print('{0},{1}'.format(2,1)) |
| |
| while True: |
| c = a + b |
| a = b |
| b = c |
| index +=1 |
| print('{0},{1}'.format(index,c)) |
| |
| if index == 101: |
| break |
pip 简单使用
| |
| ~ ➤ cat ~/.pip/pip.conf |
| [global] |
| index-url=http://mirrors.aliyun.com/pypi/simple |
| trusted-host=mirrors.aliyun.com |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
猜数字的游戏
| NUM = 35 |
| |
| count = 0 |
| |
| while count < 3: |
| user_input = int(input('请你输入一个数字: ')) |
| if user_input == NUM : |
| print('你猜对了') |
| break |
| elif user_input < NUM: |
| print('你输入的数比这个数要小') |
| else: |
| print('你输入的数比这个要大') |
| count +=1 |
| else: |
| print('你已经超过了三次机会') |
不用变量时,把变量放空
| for _ in range(0,3): |
| user_input = int(input('请你输入一个数字: ')) |
| if user_input == NUM : |
| print('你猜对了') |
| break |
| elif user_input < NUM: |
| print('你输入的数比这个数要小') |
| else: |
| print('你输入的数比这个要大') |
| count +=1 |
| else: |
| print('你已经超过了三次机会') |
打印扬辉三角
python 中求阶乘的方法
| import math |
| math.factorial(5) # 5 的阶乘 |
| import math |
| |
| lines = 10 |
| |
| for n in range(0,lines): |
| if n == 0: |
| for _ in range(0, lines // 2): |
| print(' ',end=' ') |
| print(1) |
| else: |
| for _ in range(0,lines //2): |
| print(' ',end=' ') |
| |
| for m in range(0, n+1): |
| num = math.factorial(n) //(math.factorial(m) * math.factorial(n-m)) |
| print(num, end=' ') |
| print() |
| host = {"host%d"%i: "192.168.1.%d"%i for i in range(2,11)} |
| for i in host.values(): |
| print (i) |
| |
| lst = list(range(1,101)) |
| lst[0::2] [::-1] |
| lst[1::2] [::-1] |
python 与用户交互输入位数生成若干随机数,求若干随机数的最大值,最小值
| |
| from fabric.colors import red,blue,green,cyan |
| import random |
| |
| |
| a=int(input(red('您要获取多少个随机数: '))) |
| b=int(input(red('您要获取的随机数位数: '))) + 1 |
| |
| num_two = [] |
| for i in range(int(a)): |
| num = random.randrange(0,b) |
| print(cyan('第') + blue(i + 1) + cyan('个随机数为'),blue(num)) |
| num_two.append(num) |
| print(cyan('您获取了') + blue(i + 1) + cyan('个随机数')) |
| print(cyan('您的第') + blue(num_two.index(max(num_two)) +1 ) + cyan('个随机数为最大值:'), green(max(num_two))) |
| print(cyan('您的第') + blue(num_two.index(min(num_two)) +1 ) + cyan('个随机数为最小值:'), green(min(num_two))) |
方法二
| #!/usr/bin/env python3 |
| # -*- coding: utf-8 -*- |
| from fabric.colors import red,blue,green,cyan |
| import random |
| a=int(input(red('您要获取多少个随机数: '))) |
| b=int(input(red('您要獲取的隨機數範圍: '))) + 1 |
| range_init = list(range(1,int(a)+1)) |
| print (range_init) |
| num_two = [] |
| for i in range_init: |
| num = random.randrange(0,b) |
| num = random.randrange(0,b) |
| |
| print(cyan('第') + blue(i) + cyan('个随机数为'),blue(num)) |
| num_two.append(num) |
| print(cyan('您获取了') + blue(i) + cyan('个随机数')) |
| print(cyan('您的第') + blue(num_two.index(max(num_two))+1) + cyan('个随机数为最大值:'), green(max(num_two))) |
| print(cyan('您的第') + blue(num_two.index(min(num_two))+1) + cyan('个随机数为最小值:'), green(min(num_two))) |